[국비지원교육] [패스트캠퍼스] React 강의 6주차 (1) -JS 데이터 (Javascript)
[Javascript] 데이터 data
- 문자
- 숫자와 수학
- 배열
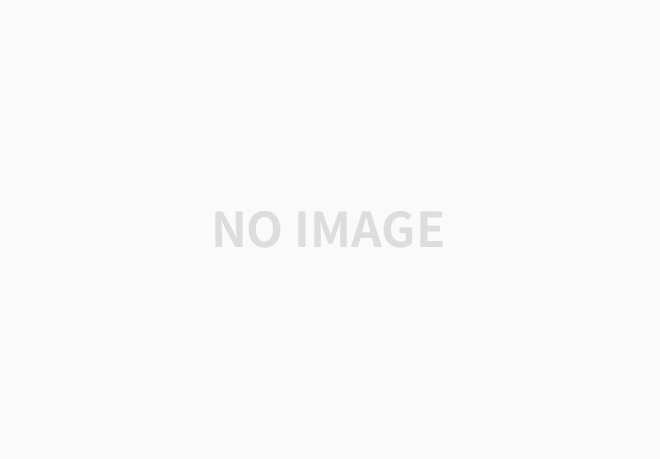
1. 문자
String 전역 객체는 문자열의 생성자 입니다.
1) String.prototype.indexOf()
indexOf() 메서드는 호출한 String 객체에서 주어진 값과 일치하는 첫 번째 인덱스를 반환합니다. 일치하는 값이 없으면 -1을 반환합니다.
searchValue
찾으려는 문자열. 아무 값도 주어지지 않으면 문자열 "undefined"를 찾으려는 문자열로 사용합니다.
searchValue의 첫 번째 등장 인덱스. 찾을 수 없으면 -1.
2) String.prototype.slice()
slice() 메소드는 문자열의 일부를 추출하면서 새로운 문자열을 반환합니다.
3) String.prototype.replace()
replace() 메서드는 어떤 패턴에 일치하는 일부 또는 모든 부분이 교체된 새로운 문자열을 반환합니다.
4) String.prototype.match()
match() 메서드는 문자열이 정규식과 매치되는 부분을 검색합니다.
5) String.prototype.trim()
trim() 메서드는 문자열 양 끝의 공백을 제거합니다.
const paragraph = 'The quick brown fox jumps over the lazy dog. If the dog barked, was it really lazy?';
const searchTerm = 'dog';
const indexOfFirst = paragraph.indexOf(searchTerm);
console.log(`The index of the first "${searchTerm}" from the beginning is ${indexOfFirst}`);
// expected output: "The index of the first "dog" from the beginning is 40"
console.log(`The index of the 2nd "${searchTerm}" is ${paragraph.indexOf(searchTerm, (indexOfFirst + 1))}`);
// expected output: "The index of the 2nd "dog" is 52"
const result = 'Hello World!'.indexOf('adlog')
console.log(result) // -1 일치하는 값이 없음.
const str = '123'
console.log(('01 23').length) //5
console.log(str.indexOf('adlog') !== -1) //false
console.log(str.slice(0, 2)) //12
console.log(str.replace('1','adlog')) //adlog23
const mail = '123@gmail.com'
console.log(mail.match(/.+(?=@)/)) //['123', index: 0, input: '123@gmail.com', groups: undefined]
console.log(mail.match(/.+(?=@)/)[0]) //123
const str_trim = ' adlog '
console.log(str_trim.trim()) //adlog 공백제거
2. 숫자와 수학
1) Number.prototype.toFixed()
toFixed() 메서드는 숫자를 고정 소수점 표기법(fixed-point notation)으로 표시합니다.
2) Number.parseInt()
Number.parseInt() 메서드는 문자열 인자를 파싱하여 특정 진수(수의 진법 체계에서 기준이 되는 값)의 정수를 반환합니다.
3) Number.parseFloat()
Number.parseFloat() 메서드는 주어진 값을 필요한 경우 문자열로 변환한 후 부동소수점 실수로 파싱해 반환합니다. 숫자를 파싱할 수 없는 경우 NaN을 반환합니다.
4) Number.prototype.toFixed()
toFixed() 메서드는 숫자를 고정 소수점 표기법(fixed-point notation)으로 표시합니다.
const pi = 3.141592653
console.log(pi) //3.141592653
const str = pi.toFixed(2)
console.log(str) //3.14
console.log(typeof str) //string
const integer = parseInt(str)
const float = parseFloat(str)
console.log(integer) //3
console.log(float) //3.14
console.log(typeof integer, typeof float) //number number
Math
**Math**는 수학적인 상수와 함수를 위한 속성과 메서드를 가진 내장 객체입니다. 함수 객체가 아닙니다.
Math는 Number 자료형만 지원하며 BigInt와는 사용할 수 없습니다.
1) Math.abs()
Math.abs() 함수는 주어진 숫자의 절대값을 반환합니다. x가 양수이거나 0이라면 x를 리턴하고, x가 음수라면 x의 반대값, 즉 양수를 반환합니다.
2) Math.ceil()
Math.ceil() 함수는 주어진 숫자보다 크거나 같은 숫자 중 가장 작은 숫자를 integer 로 반환합니다.
3) Math.floor()
Math.floor() 함수는 주어진 숫자와 같거나 작은 정수 중에서 가장 큰 수를 반환합니다.
4) Math.round()
Math.round() 함수는 입력값을 반올림한 수와 가장 가까운 정수 값을 반환합니다.
6) Math.random()
Math.random() 함수는 0 이상 1 미만의 구간에서 근사적으로 균일한(approximately uniform) 부동소숫점 의사난수를 반환하며, 이 값은 사용자가 원하는 범위로 변형할 수 있다. 난수 생성 알고리즘에 사용되는 초기값은 구현체가 선택하며, 사용자가 선택하거나 초기화할 수 없다.
function difference(a, b) {
return Math.abs(a - b);
}
console.log(difference(3, 5));// expected output: 2
console.log(difference(5, 3));// expected output: 2
console.log(difference(1.23456, 7.89012));// expected output: 6.6555599999999995
console.log('abs: ', Math.abs(-12)) // 12 양수
console.log('min: ', Math.min(2, 8)) // 2 작은수
console.log('max: ', Math.max(2, 8)) // 8 큰수
console.log('ceil: ', Math.ceil(3.14)) // 4 올림
console.log('floor: ', Math.floor(3.14)) // 3 내림
console.log('round: ', Math.round(3.14)) // 3 반내림
console.log('random: ', Math.random()) // 0.9438494951620242 랜덤숫자
3. 배열(Array)
JavaScript Array 클래스는 리스트 형태의 고수준 객체인 배열을 생성할 때 사용하는 전역 객체입니다.
1) Array.length
Array 인스턴스의 length 속성은 배열의 길이를 반환합니다. 반환값은 부호 없는 32비트 정수형이며, 배열의 최대 인덱스보다 항상 큽니다. length 속성에 값을 설정할 경우 배열의 길이를 변경합니다.
2) Array.prototype.find()
find() 메서드는 주어진 판별 함수를 만족하는 첫 번째 요소의 값을 반환합니다. 그런 요소가 없다면 undefined를 반환합니다.
3) Array.prototype.concat()
concat() 메서드는 인자로 주어진 배열이나 값들을 기존 배열에 합쳐서 새 배열을 반환합니다.
(** 원본의 데이터 배열에는 손상이 없습니다.)
4) Array.prototype.forEach()
forEach() 메서드는 주어진 함수를 배열 요소 각각에 대해 실행합니다.
5) Array.prototype.map()
map() 메서드는 배열 내의 모든 요소 각각에 대하여 주어진 함수를 호출한 결과를 모아 새로운 배열을 반환합니다.
const numbers = [1, 2, 3, 4]
const fruits = ['Apple', 'Banana', 'Cherry']
console.log(numbers) //(4) [1, 2, 3, 4]
console.log(numbers[1]) // 2
console.log(fruits) //(3) ['Apple', 'Banana', 'Cherry']
console.log(fruits[2]) // Cherry
console.log([1, 2].length) //2
console.log([].length) // 0 빈배열
//find()
const array1 = [5, 12, 8, 130, 44];
const found = array1.find(element => element > 10);
console.log(found)// 12 > 10보다큰 첫번째 값
///concat()
console.log(numbers.concat(fruits)) //(7) [1, 2, 3, 4, 'Apple', 'Banana', 'Cherry']
///forEach()
fruits.forEach(function (element, index, array){
console.log(element, index, array)
})
//Apple 0 (3) ['Apple', 'Banana', 'Cherry']
//Banana 1 (3) ['Apple', 'Banana', 'Cherry']
//Cherry 2 (3) ['Apple', 'Banana', 'Cherry']
fruits.forEach(function (fruit, i){
console.log(fruit, i)
})
//Apple-0
//Banana-1
//Cherry-2
const array1 = ['a', 'b', 'c'];
array1.forEach(element => console.log(element));
// "a"
// "b"
// "c"
//map()
const a = fruits.forEach(function (fruit, index){
console.log(`${fruit}-${index}`)
})
const a_1 = fruits.forEach((fruit, index) => { //화상표함수로 표현(this차이)
console.log(`${fruit}-${index}`)
})
console.log(a)
//Apple 0
//Banana 1
//Cherry 2
//undefined
const b = fruits.map(function (fruit, index){
return `${fruit}-${index}`
})
console.log(b) //(3) ['Apple-0', 'Banana-1', 'Cherry-2']
// 리터럴 방식
const c = fruits.map(function (fruit, index){
return {
id: index,
name: fruit
}
})
const c_1 = fruits.map( (fruit, index) => ({
id: index,
name: fruit
}))
console.log(c)
// (3) [{…}, {…}, {…}]
// 0: {id: 0, name: 'Apple'}
// 1: {id: 1, name: 'Banana'}
// 2: {id: 2, name: 'Cherry'}
// length: 3
// [[Prototype]]: Array(0)
Array.prototype.filter()
filter() 메서드는 주어진 함수의 테스트를 통과하는 모든 요소를 모아 새로운 배열로 반환합니다.
Array.prototype.findIndex()
findIndex() 메서드는 주어진 판별 함수를 만족하는 배열의 첫 번째 요소에 대한 인덱스를 반환합니다. 만족하는 요소가 없으면 -1을 반환합니다.
const a = numbers.map(number => {
return number < 3
})
console.log(a) //(4) [true, true, false, false]
const b = numbers.filter(number => {
return number < 3
})
console.log(b) //(2) [1, 2]
const c = fruits.find(fruit => {
return /^B/.test(fruit)
})
console.log(c) //Banana
const d = fruits.findIndex(fruit => {
return /^C/.test(fruit)
})
console.log(d) //2
Array.prototype.includes()
includes() 메서드는 배열이 특정 요소를 포함하고 있는지 판별합니다.
const a = numbers.includes(3)
console.log(a) // true
const b = fruits.includes('adlog')
console.log(b) //false
Array.prototype.push()
push() 메서드는 배열의 끝에 하나 이상의 요소를 추가하고, 배열의 새로운 길이를 반환합니다.
Array.prototype.unshift()
unshift() 메서드는 새로운 요소를 배열의 맨 앞쪽에 추가하고, 새로운 길이를 반환합니다.
Array.prototype.reverse()
reverse() 메서드는 배열의 순서를 반전합니다. 첫 번째 요소는 마지막 요소가 되며 마지막 요소는 첫 번째 요소가 됩니다.
Array.prototype.slice()
slice() 메서드는 어떤 배열의 begin 부터 end 까지(end 미포함)에 대한 얕은 복사본을 새로운 배열 객체로 반환합니다. 원본 배열은 바뀌지 않습니다.
//***원본 데이터가 수정됩니다.
numbers.push(5) //가장 뒤쪽에 데이터가 삽입
console.log(numbers) //(5) [1, 2, 3, 4, 5]
numbers.unshift(0) //첫번째에 데이터가가 삽입
console.log(numbers) //(6) [0, 1, 2, 3, 4, 5]
numbers.reverse()
fruits.reverse()
console.log(numbers) //(4) [4, 3, 2, 1]
console.log(fruits) //(3) ['Cherry', 'Banana', 'Apple']
numbers.splice(2, 1)
console.log(numbers) //(3) [1, 2, 4]
numbers.splice(2, 1, 999)
console.log(numbers) //(4) [1, 2, 999, 4]
[참고 사이트]
1. JavaScript- String
https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/String
String - JavaScript | MDN
String 전역 객체는 문자열(문자의 나열)의 생성자입니다.
developer.mozilla.org
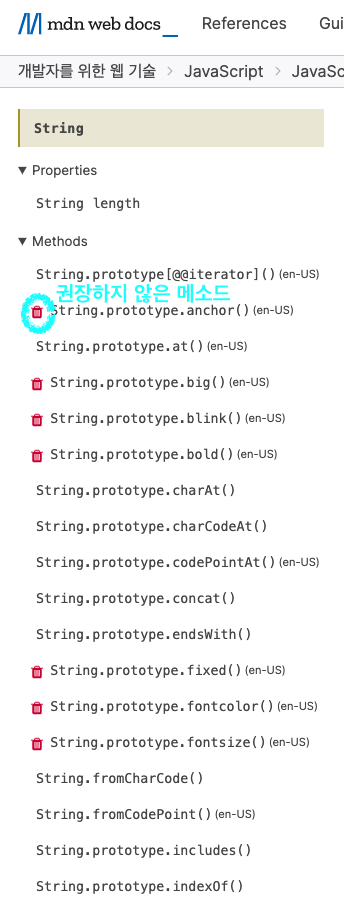
2. 배열
https://developer.mozilla.org/ko/docs/Web/JavaScript/Reference/Global_Objects/Array
Array - JavaScript | MDN
JavaScript Array 클래스는 리스트 형태의 고수준 객체인 배열을 생성할 때 사용하는 전역 객체입니다.
developer.mozilla.org
[Javascript] 데이터 data
- 문자
- 숫자와 수학
- 배열
'스터디 > React' 카테고리의 다른 글
[React] 강의 6주차 (3) -JS 데이터 (Javascript) (0) | 2022.12.07 |
---|---|
[React] 강의 6주차 (2) -JS 데이터 (Javascript) (0) | 2022.11.27 |
[React] 강의 5주차 (2) -JS (Javascript) 함수 (0) | 2022.11.16 |
[React] 강의 5주차 (1) -JS (Javascript) (0) | 2022.11.15 |
[React] 강의 4주차 (3) -JS (Node.js / NVM, NPM) (0) | 2022.11.08 |