[국비지원교육] [패스트캠퍼스] React 강의 7주차 (1) - React 구현 전 확인사항
[Javascript] React
- Dom Render
- Webpck, babel 세팅
- create Element
- @Jsx
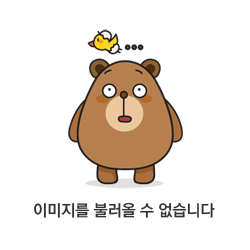
1. DomRander
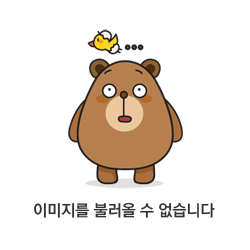
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>react강의</title>
</head>
<body>
<div id="root"></div>
<script src="app.js" type="module"></script>
</body>
</html>
[app.js]
function createDOM(vdom){
if(typeof vdom === 'string') {
return document.createTextNode(vdom);
}
const element = document.createElement(vdom.tag);
vdom.children
.map(createDOM)
.forEach(element.appendChild.bind(element));
return element;
}
const vdom = {
tag: 'p',
props: [],
children: [
{
tag: 'h1',
props: {},
children: ["React 만들기"],
},
{
tag: 'ul',
props: {},
children: [
{
tag: 'li',
props: {},
children: ["첫 번째 아이템"]
},
{
tag: 'li',
props: {},
children: ["두 번째 아이템"]
},
{
tag: 'li',
props: {},
children: ["세 번째 아이템"]
}
]
}
],
};
document
.querySelector("#root")
.appendChild(createDOM(vdom));
2. Webpck, babel 세팅
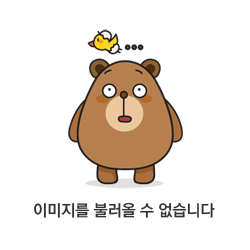
oddm@oddmui-MacBookPro react_test % npm init -y
npm install webpack cli --save-dev // 웹팩 설치
ddm@oddmui-MacBookPro react_test % npm install webpack-dev-server babel-loader @babel/core @babel/preset-env @babel/preset-react html-webpack-plugin --save-dev
//바벨 설치
> webpack.config.js 파일 만들기
const HtmlWebpackPlugin = require('html-webpack-plugin');
const path = require('path');
module.exports = {
mode: 'development',
entry: './app.js',
output: {
path: path.resolve(__dirname, 'dist'),
filename: 'bundle.js'
},
devServer: {
compress: true,
port: 9999,
},
module: {
rules: [
{
test: /\.js$/, //확장자가 js 파일들은 아래 바벨로더에 넣어줘~
exclude: /node_modules/,
use: {
loader: 'babel-loader',
options: {
presets: ["@babel/preset-env", "@babel/preset-react"]
}
}
}
]
},
plugins: [
new HtmlWebpackPlugin({
title: 'react강의',
template: 'index.html'
})
]
}
> babel.config.js 파일 만들기
{
"presets": ["@babel/preset-env"]
}
> package.json scripts 추가
{
"name": "react_test",
"version": "1.0.0",
"description": "",
"main": "app.js",
"scripts": {
"build": "webpack",
"dev": "webpack serve",
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC",
"devDependencies": {
"@babel/core": "^7.20.5",
"@babel/preset-env": "^7.20.2",
"@babel/preset-react": "^7.18.6",
"babel-loader": "^9.1.0",
"cli": "^1.0.1",
"html-webpack-plugin": "^5.5.0",
"webpack": "^5.75.0",
"webpack-cli": "^5.0.1",
"webpack-dev-server": "^4.11.1"
}
}
> npm run dev (잘동작하는 것을 확인합니다. 오류사항은 해당 라인 고치면 됩니다!)
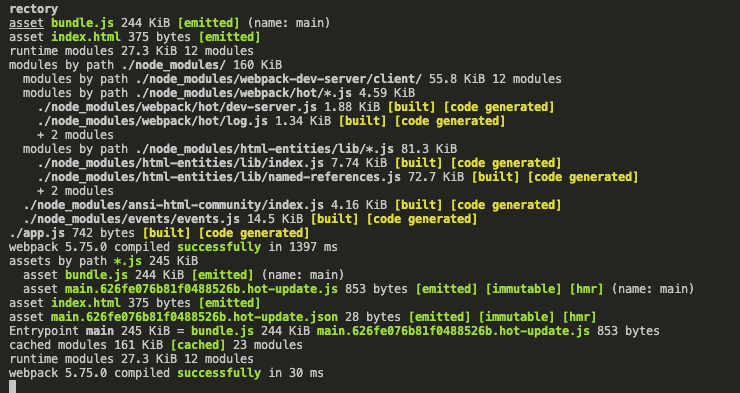
>npm run build (dist 폴더가 생겨납니다.)
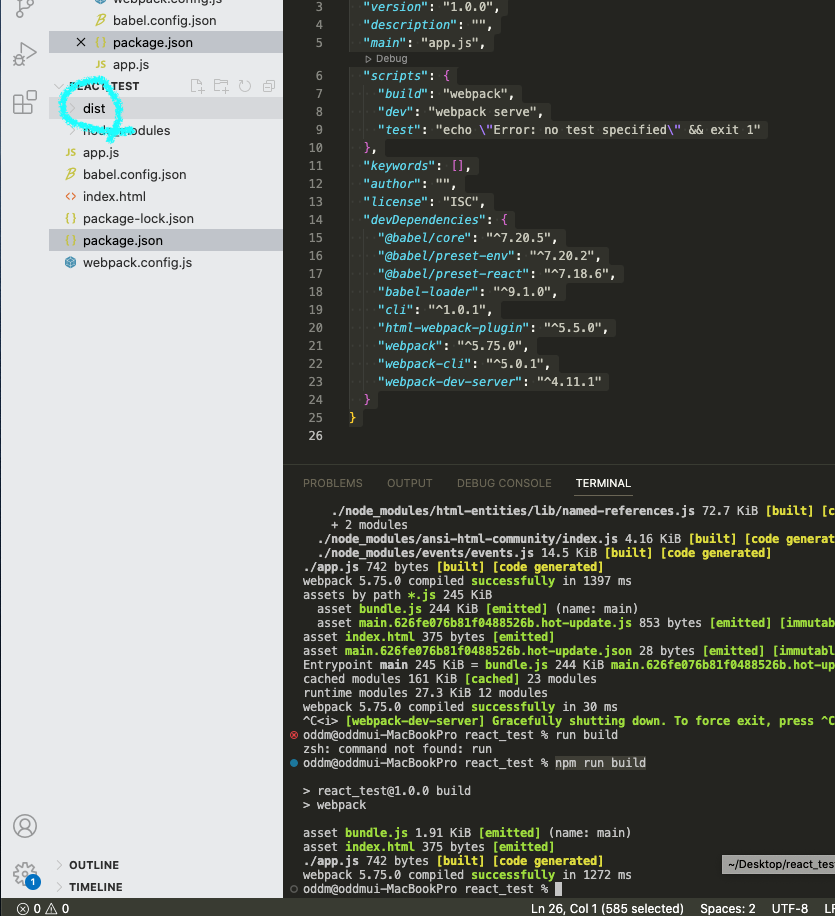
> html 파일에서 <script src="./app.js" type="module"></script> 삭제후 ,
> npm run dev
<!DOCTYPE html>
<html lang="ko">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>react강의</title>
</head>
<body>
<div id="root"></div>
</body>
</html>
> src 폴더를 만들어서 아래와 같이 수정해 줍니다. > package.json entry 출력에 루트를 수정후에
> npm run dev로 확인해줍니다.
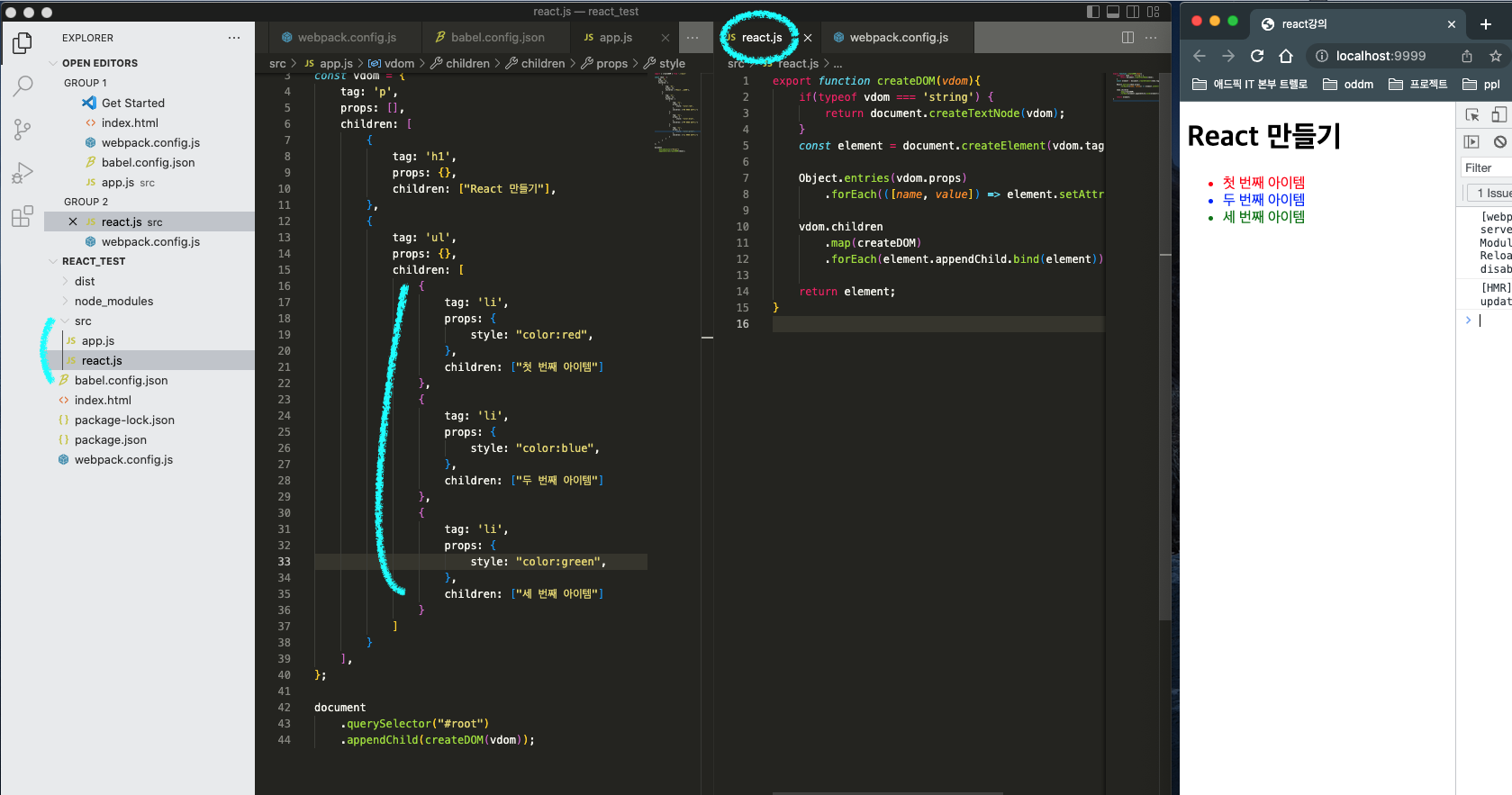
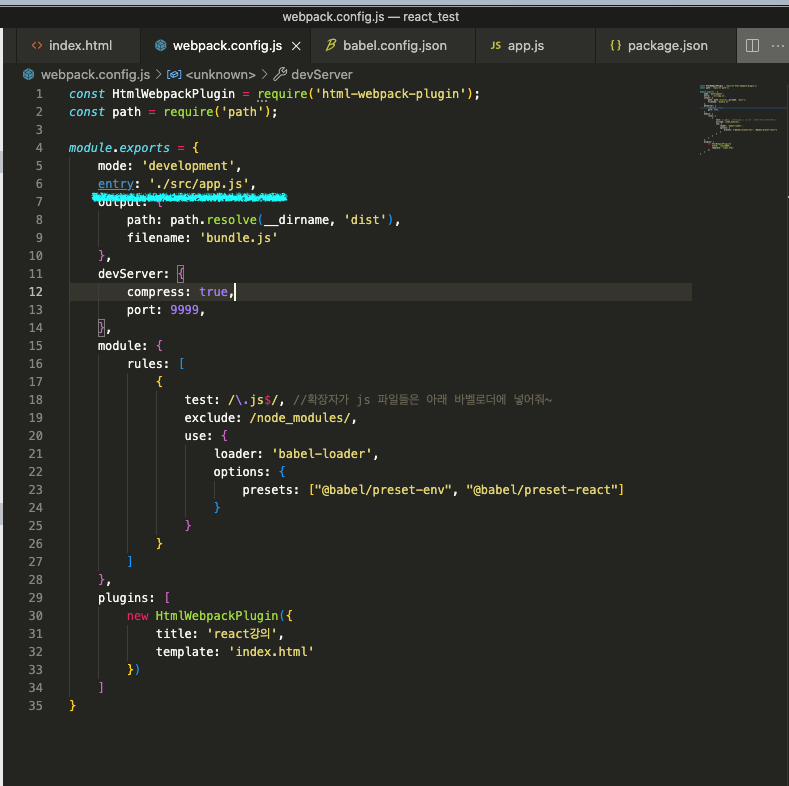
> 구조정리
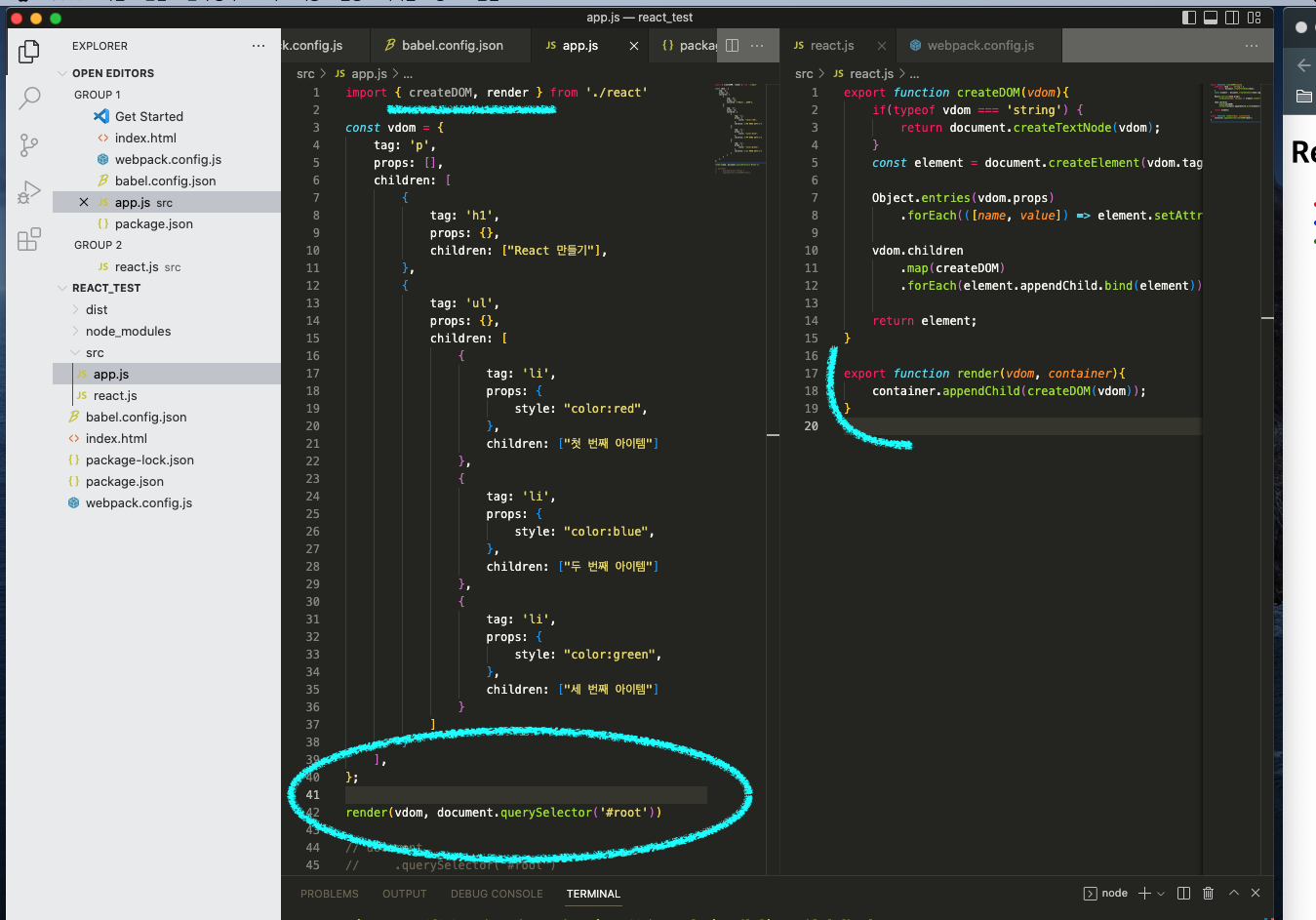
3.create Element (사용성 개선) > 코드량을 줄였다
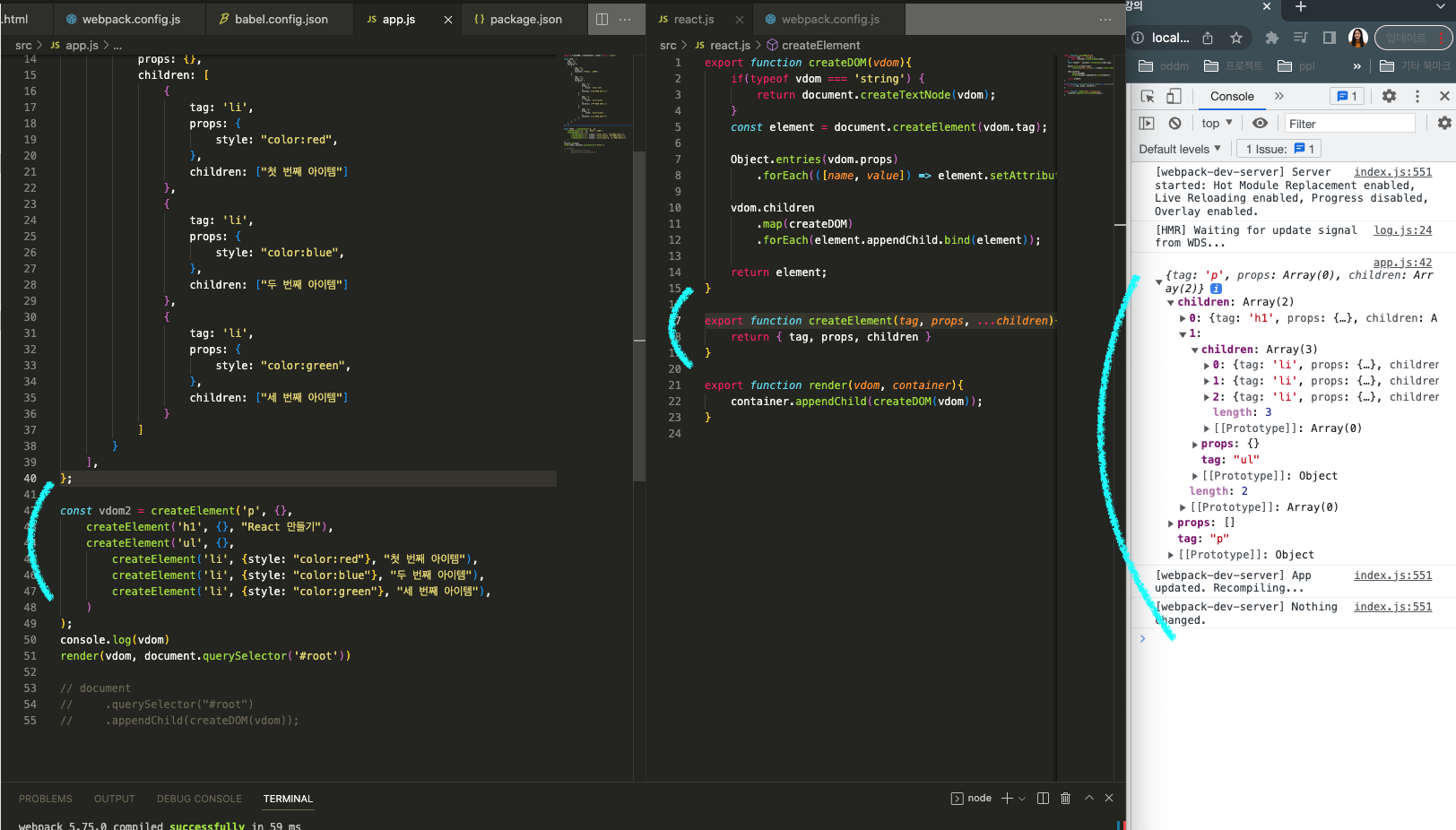
const vdom2 = createElement('p', {},
createElement('h1', {}, "React 만들기"),
createElement('ul', {},
createElement('li', {style: "color:red"}, "첫 번째 아이템"),
createElement('li', {style: "color:blue"}, "두 번째 아이템"),
createElement('li', {style: "color:green"}, "세 번째 아이템"),
)
);
console.log(vdom)
export function createElement(tag, props, ...children){
return { tag, props, children }
}
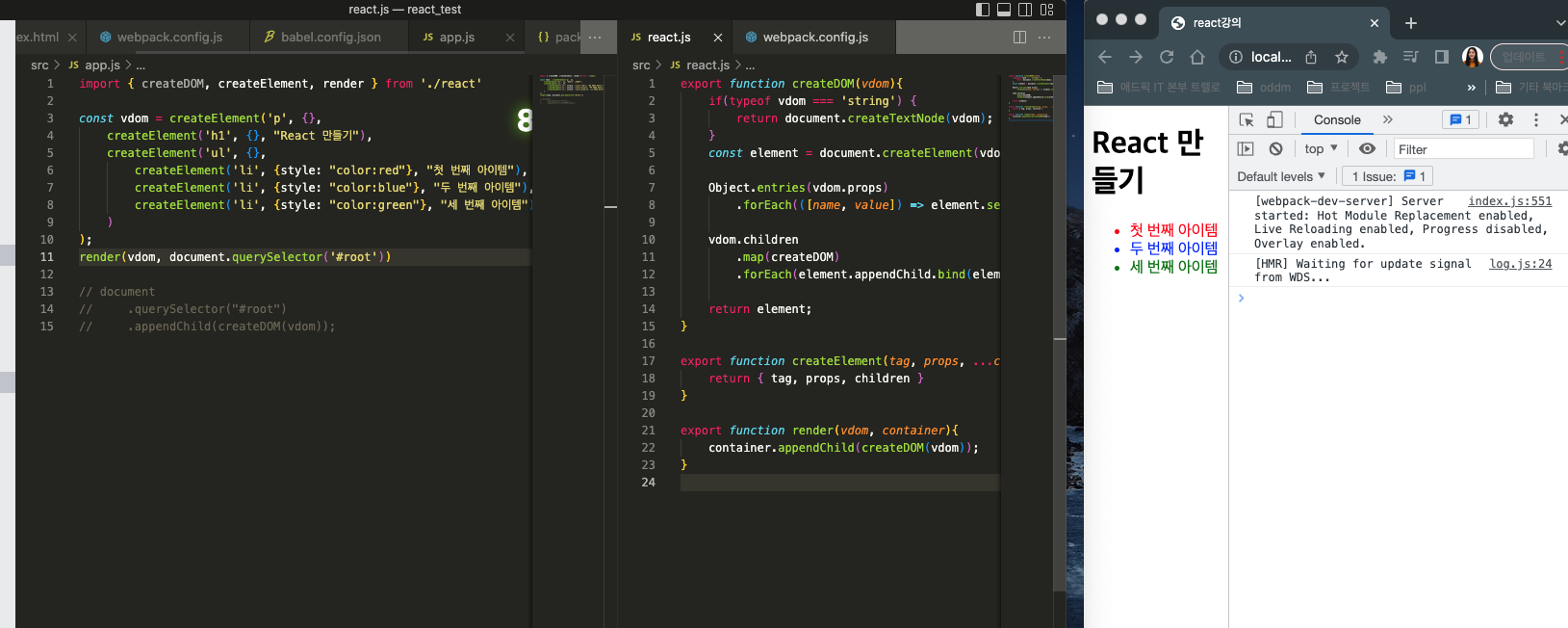
4. @Jsx
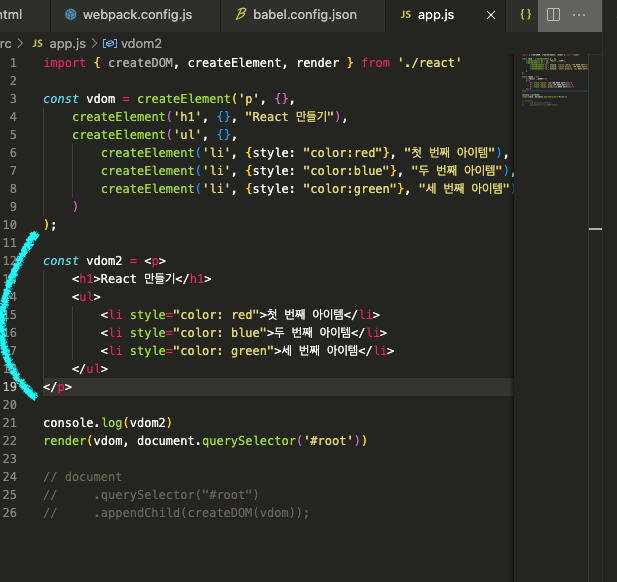
>bundle.js:299 Uncaught ReferenceError: React is not defined
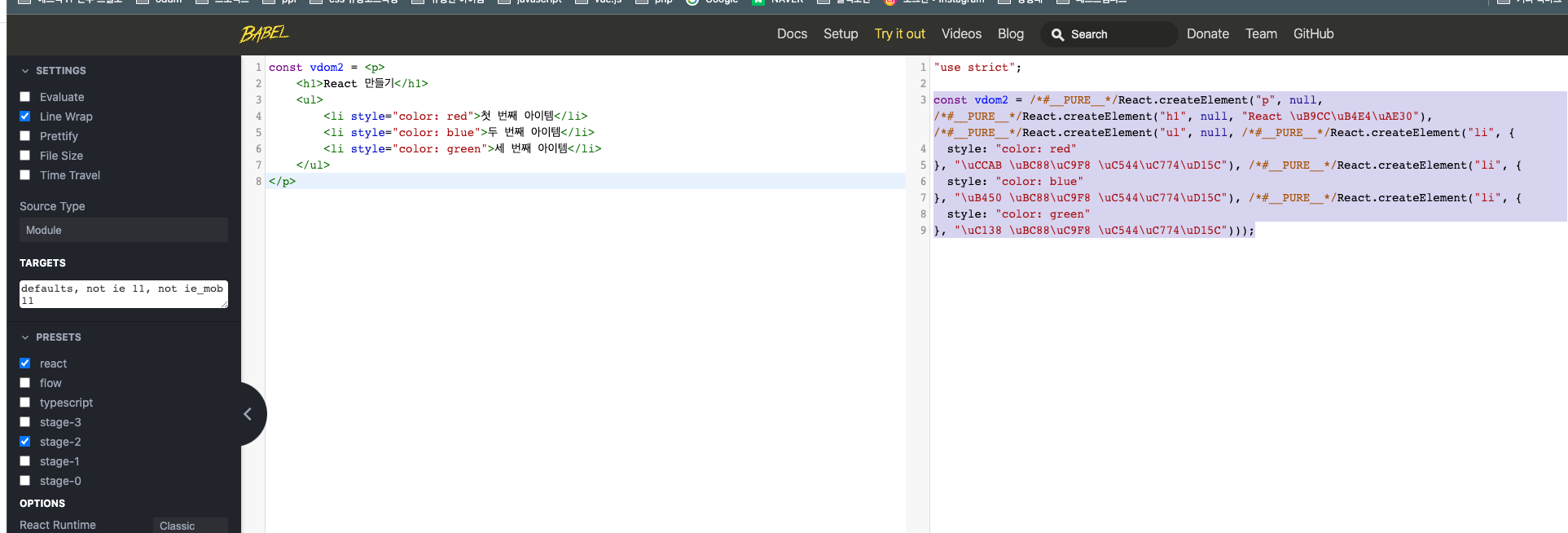
> 수정
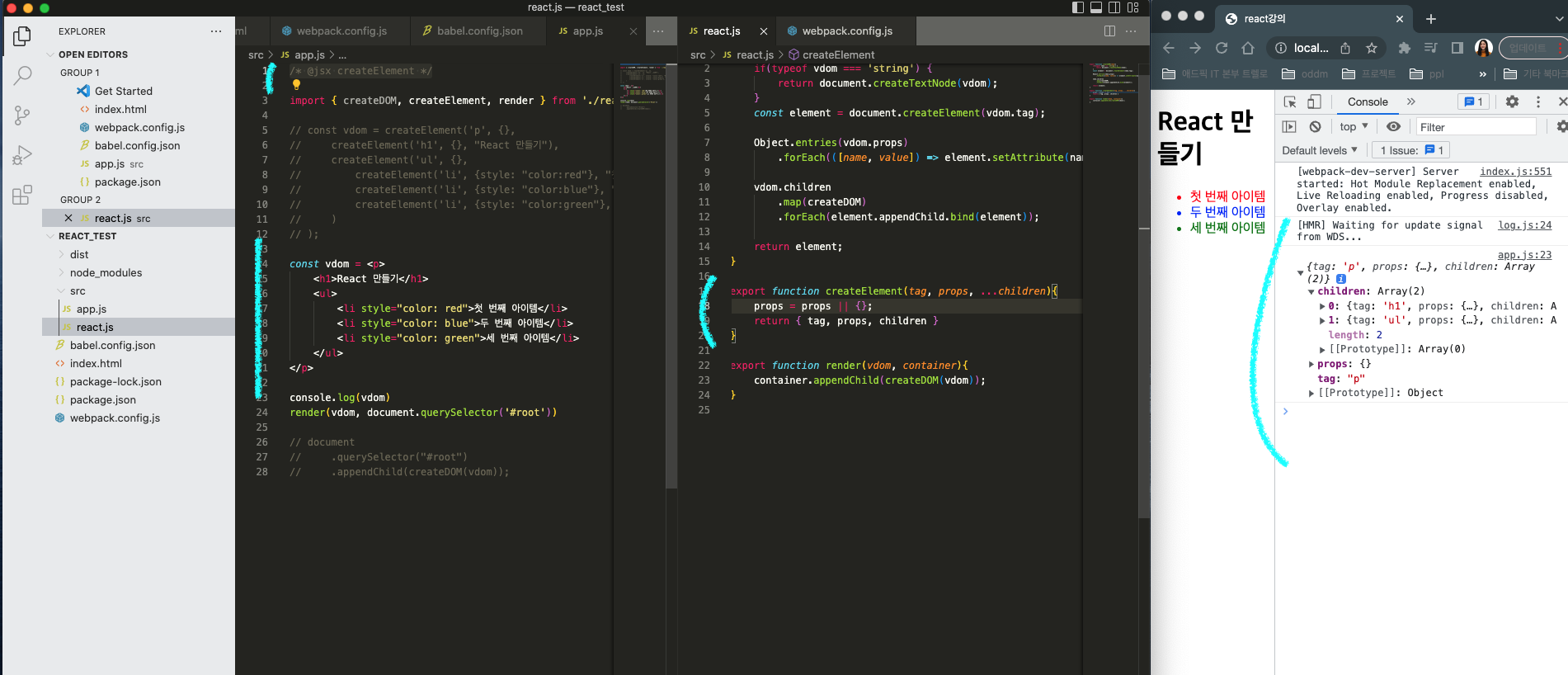
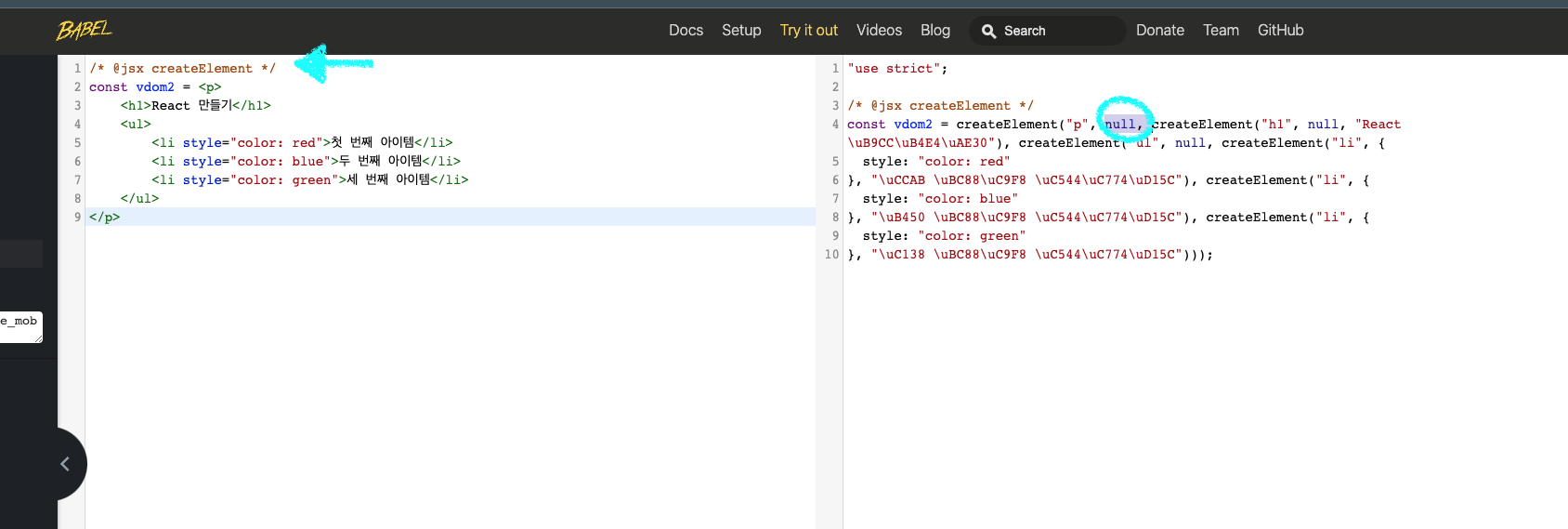
[참고사이트]
1) react github
https://github.com/facebook/react/
GitHub - facebook/react: A declarative, efficient, and flexible JavaScript library for building user interfaces.
A declarative, efficient, and flexible JavaScript library for building user interfaces. - GitHub - facebook/react: A declarative, efficient, and flexible JavaScript library for building user interf...
github.com
2) expressjs
Node.js를 위한 빠르고 개방적인 간결한 웹 프레임워크
Express - Node.js 웹 애플리케이션 프레임워크
Node.js를 위한 빠르고 개방적인 간결한 웹 프레임워크 $ npm install express --save
expressjs.com
3) webpack
webpack
웹팩은 모듈 번들러입니다. 주요 목적은 브라우저에서 사용할 수 있도록 JavaScript 파일을 번들로 묶는 것이지만, 리소스나 애셋을 변환하고 번들링 또는 패키징할 수도 있습니다.
webpack.kr
4) babel
Babel · The compiler for next generation JavaScript
The compiler for next generation JavaScript
babeljs.io
[Javascript] React
- Dom Render
- Webpck, babel 세팅
- create Element
- @Jsx
'스터디 > React' 카테고리의 다른 글
[React] 강의 8주차 (1) - React _ Redux 만들기 (0) | 2023.01.13 |
---|---|
[React] 강의 7주차 (2) - React 원리 (0) | 2022.12.08 |
[React] 강의 6주차 (3) -JS 데이터 (Javascript) (0) | 2022.12.07 |
[React] 강의 6주차 (2) -JS 데이터 (Javascript) (0) | 2022.11.27 |
[React] 강의 6주차 (1) -JS 데이터 (Javascript) (0) | 2022.11.17 |